Angular use observables to pass values that change over time. A common example is listening to the current path of the app:
this.router.events.subscribe((event) => {
if (event instanceof NavigationEnd) {
debug('this.router.url',this.router.url);
}
})
If you call a .subscribe method like the example above, you can cause a memory leak. You need to be sure to unsubscribe when your UI is unloaded.
Single Subscription
If your component only uses one subscription, you can add a subscription property to your UI:
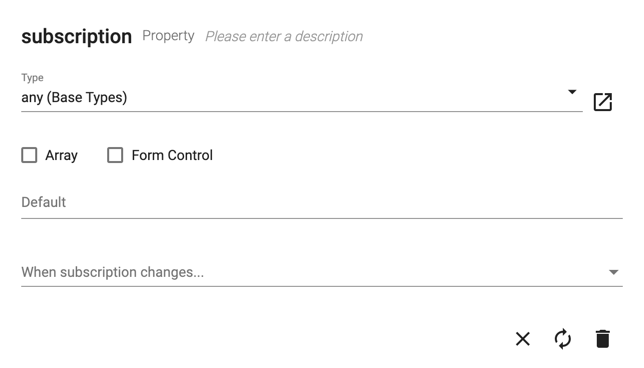
And add an unsubscribe UI method to clean it up:
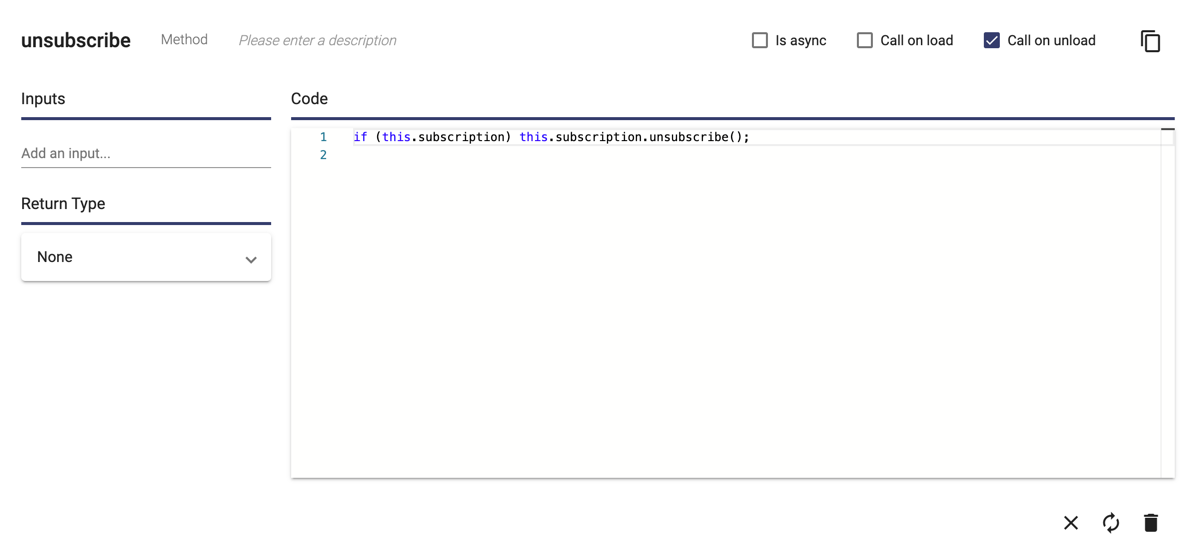
Multiple Subscriptions
If your app uses more than one subscription, you can make the property subscriptions:
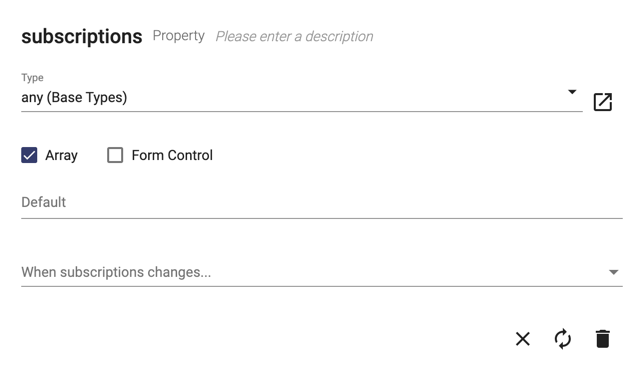
And subscribe to the observable like this:
this.subscriptions.push(
this.router.events.subscribe((event) => {
if (event instanceof NavigationEnd) {
debug('this.router.url',this.router.url);
}
})
);
And make the unsubscribe method like this:
while (this.subscriptions.length > 0) {
this.subscriptions.pop().unsubscribe();
}
You can use the multiple pattern for a single subscription as well. You never know when you might need to add another!